python 小技巧(python tricks)
不积跬步无以至千里
Github地址:https://github.com/vimiix/python_tricks
Slide链接:http://vimiix.com/python_tricks
人生苦短,我用Python
Life is short, use Python.
Python之禅
import this
'''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
...
'''
多种方法来验证多条件语句
x, y, z = 0, 1, 0
if x == 1 or y == 1 or z == 1:
print('passed')
if 1 in (x, y, z):
print('passed')
# These only test for truthiness:
if x or y or z:
print('passed')
if any((x, y, z)):
print('passed')
函数参数解包
# Function argument unpacking
def myfunc(x, y, z):
print(x, y, z)
tuple_vec = (1, 0, 1)
dict_vec = {'x': 1, 'y': 0, 'z': 1}
myfunc(*tuple_vec)
# 1, 0, 1
myfunc(**dict_vec)
# 1, 0, 1
使用timeit模块来测试函数执行效率
import timeit
rt = timeit.timeit('"-".join(str(n) for n in range(100))',
number=10000)
print(rt)
# 0.252703905106
rt = timeit.timeit('"-".join([str(n) for n in range(100)])',
number=10000)
print(rt)
# 0.234980106354
rt = timeit.timeit('"-".join(map(str, range(100)))',
number=10000)
print(rt)
# 0.152318954468
合并两个字典在不同版本中的写法
x = {'a': 1, 'b': 2}
y = {'b': 3, 'c': 4}
# Python 3.5+
z = {**x, **y}
print(z)
# {'c': 4, 'a': 1, 'b': 3}
# Python 2.x
z = dict(x, **y)
print(z)
# {'a': 1, 'c': 4, 'b': 3}
巧用json模块更舒服的打印字典
# 标准打印出来,对于阅读不是很友好
my_mapping = {'a': 23, 'b': 42, 'c': 0xc0ffee}
print(my_mapping)
# {'b': 42, 'c': 12648430. 'a': 23} # đ
# 使用json可以更好的展示
import json
print(json.dumps(my_mapping, indent=4, sort_keys=True))
# {
# "a": 23,
# "b": 42,
# "c": 12648430
# }
通过字典的值(value)排序输出
# How to sort a Python dict by value
xs = {'a': 4, 'b': 3, 'c': 2, 'd': 1}
print(sorted(xs.items(), key=lambda x: x[1]))
# [('d', 1), ('c', 2), ('b', 3), ('a', 4)]
# Or:
import operator
print(sorted(xs.items(), key=operator.itemgetter(1)))
# [('d', 1), ('c', 2), ('b', 3), ('a', 4)]
字典的get用法和默认值
name_for_userid = {
382: "Alice",
590: "Bob",
951: "Dilbert",
}
def greeting(userid):
return "Hi %s!" % name_for_userid.get(userid, "there")
print(greeting(382))
# "Hi Alice!"
print(greeting(2333333))
# "Hi there!"用namedtuple
来代替简单的类
from collections import namedtuple
Car = namedtuple('Car', 'color mileage')
# 实例化一个Car,并像类一样使用
my_car = Car('red', 3812.4)
print(my_car.color)
# 'red'
print(my_car.mileage)
# 3812.4
print(my_car)
# Car(color='red' , mileage=3812.4)
# 就像元组一样,namedtuple也不可更改:
my_car.color = 'blue'
# AttributeError: "can't set attribute"
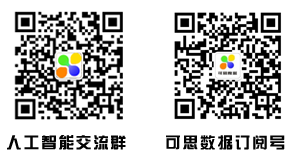
时间:2018-10-09 22:38 来源: 转发量:次
声明:本站部分作品是由网友自主投稿和发布、编辑整理上传,对此类作品本站仅提供交流平台,转载的目的在于传递更多信息及用于网络分享,并不代表本站赞同其观点和对其真实性负责,不为其版权负责。如果您发现网站上有侵犯您的知识产权的作品,请与我们取得联系,我们会及时修改或删除。
相关文章:
- [数据挖掘]底层I/O性能大PK:Python/Java被碾压,Rust有望取代
- [数据挖掘]大数据分析的技术有哪些?
- [数据挖掘]大数据分析会遇到哪些难题?
- [数据挖掘]RedMonk语言排行:Python力压Java,Ruby持续下滑
- [数据挖掘]不得了!Python 又爆出重大 Bug~
- [数据挖掘]TIOBE 1 月榜单:Python年度语言四连冠,C 语言再次
- [数据挖掘]TIOBE12月榜单:Java重回第二,Python有望四连冠年度
- [数据挖掘]这个可能打败Python的编程语言,正在征服科学界
- [数据挖掘]2021年编程语言趋势预测:Python和JavaScript仍火热,
- [数据挖掘]Spark 3.0重磅发布!开发近两年,流、Python、SQL重
相关推荐:
网友评论: